Realization of a Multiple Selection (Multiple Choice)
This article is about selecting multiple options through user input or dialog boxes. The primary topic is a method for command files or scripts. In addition to console input, GUI options are also discussed. Procedures are presented for both environments, based on the means available by default in Windows.
Windows Console
In command files, the console program Choice (choice.exe) can be used:
choice /c 123 /n /m "1 = Option 1, 2 = Option 2, 3 = Option 3" echo %errorlevel%
The selection is made by pressing a valid number or letter key. The exit code corresponds to the index of the chosen option. It is 255 with invalid syntax.
However, only one option can be selected in this way.
To allow multiple options to be selected, one can use the following method:
@echo off echo Choices: echo 1. Option 1 echo 2. Option 2 echo 3. Option 3 set /p _input=Please enter the numbers of your selection (e.g. 1,3): echo %_input%
Here, multiple numbers can be entered. With regard to further processing (as well as the possibility of numerical values for more than 9 options), a comma separation is useful.
In principle, the option numbers can then simply be processed:
for %%X in (%_input%) do echo %%X
With a larger number of options, entering every single numeral becomes inconvenient, and the ability to also specify ranges like "3-5" will be desirable. Such mixed forms of selection are found in many dialogs and are therefore generally familiar to users.
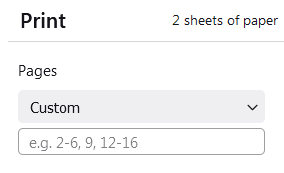
However, this makes the evaluation much more complicated. Above all, the intermediate values are to be determined for range specifications. It is certainly expedient to create a new, extended number list, which can then be processed again in a For loop.
I wrote a command file that does this task (multichoice.bat). This file is primarily for demonstration purposes. In addition to the evaluation, it contains a simple input prompt and a display of the final list of numbers. You can easily adapt the file for your purposes by adding the display and selection of the options in the desired way.
The two command files options.bat and mchoice.bat are more intended for practical use, in which the selection and processing of options (options.bat) and the input evaluation (mchoice.bat) are separated. Modifications here can be limited to the options.bat file.
In principle, the selection by entering option numbers represents a weak point, since many incorrect entries are possible. Also note that the same option numbers can be entered multiple times.
Checking for these input errors can be quite difficult and excessive. The provided command file (mchoice.bat) largely omits such checks. (Yet there's a check for i2>i1 if a range i1-i2 is specified.) Wrong numbers or letters are usually not critical. However, there are conceivable entries that cause problems during processing. If necessary, perform appropriate checks based on your own tests. For a small number of options, the range input could be omitted for simplicity.
GUI Dialogs
A multiple selection can be realized in a similar way with VBScript. The InputBox function is available for displaying and entering the options within a dialog window.
prompt = "Choices:" & vbNewLine & _ "1. Option 1" & vbNewLine & _ "2. Option 2" & vbNewLine & _ "3. Option 3" & vbNewLine & _ "Please enter the numbers of your selection (e.g. 1,3)." input = InputBox(prompt, "Selection")
As to the rest, the above applies to the procedure. The input can be evaluated using the means available in VBScript (string functions, RegExp object). An example application can be found in multichoice.vbs. Here, too, I have made an additional separation of option selection (options.vbs) and input evaluation (mchoice.vbs). Again, a mutual call takes place between both files. It would also be possible to use the mchoice.bat command file, as indicated in options.vbs. And vice versa, the options.bat console input could invoke the mchoice.vbs script.
Another solution is available via the HTML application (HTA). Put simply, this is an executable HTML file that typically combines HTML controls with VBScript or JScript functions. The executing program mshta.exe is a standard part of Windows.
For example, the dialog box could be defined like this:
<body> <p> Select the desired options: </p> <p> <input type="checkbox" name="chkOpt1"/>Option 1<br> <input type="checkbox" name="chkOpt2"/>Option 2<br> <input type="checkbox" name="chkOpt3"/>Option 3 </p> <p> <input type="button" value="OK" name="btnOK"/> <input type="button" value="Cancel" name="btnCancel"/> </p> </body>
The header of the HTA file contains VBScript procedures that handle the two button clicks:
<html> <head> <title>Multiple Choice Dialog</title> <script language="vbscript"> Sub btnOK_onClick Dim msg msg = "" If chkOpt1.checked = True Then msg = msg & "1" & vbNewLine ' process option 1 End If If chkOpt2.checked = True Then msg = msg & "2" & vbNewLine ' process option 2 End If If chkOpt3.checked = True Then msg = msg & "3" & vbNewLine ' process option 3 End If If Len(msg) = 0 Then msg = "(None)" MsgBox msg, , "Selected Options" Window.Close End Sub Sub btnCancel_onClick Window.Close End Sub </script> <hta:application> </head>
The file is available as multichoice.hta. It contains a few additional window styling instructions.
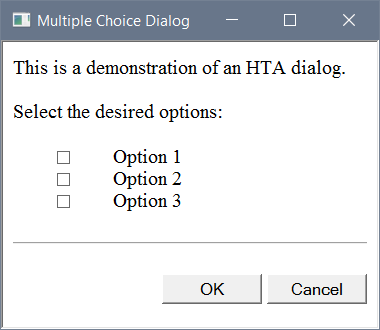
Of course, one could also use HTA to enter option numbers using the methods shown above. However, the selection using checkbox elements is certainly more intuitive for users and also has the great advantage that complicated evaluation and error checking of the input is no longer necessary.
PowerShell
With PowerShell, user input can be processed in the console window or passed to Cmd or a command file. Additionally, .NET Framework classes can be used to display GUI dialogs for input and list selection.
Examples of this are included in the PowerShell documentation, so we don't need to go into them further here (Links: Read-Host, Input box, List box).
Miscellaneous
Finally, it should be noted that selections can also be realized with VTOOL dialog windows. (Providing such GUI dialogs was one of the main reasons for developing VTool.)
However, VTool is a supplementary component. Therefore, its use requires a previous installation (object registration).
VTool supplies two different list windows that allow multiple selections. In addition, VTool makes the task dialog available, which allows selection of an option via radio buttons.
A multiple selection could be set up with the CheckList object, for example:
options = "Option 1" & vbNewLine & "Option 2" & vbNewLine & "Option 3" Set obj = CreateObject("VTool.CheckList") choice = obj.Selection(options, , "Select the desired options")
VTool.CheckList does not return a numerical list of the selected options but a total value from the powers of two of the indices. The indices here start at 0, so each index is 1 lower than the associated option number. This may sound complicated, but it's actually very easy to evaluate. For example, if a user selects the first and third items in the list, the function returns 5 (20 + 22). The corresponding values are checked for evaluation:
msg = "" If choice And 2^0 Then msg = msg & "1" & vbNewLine ' process option 1 End If If choice And 2^1 Then msg = msg & "2" & vbNewLine ' process option 2 End If If choice And 2^2 Then msg = msg & "3" & vbNewLine ' process option 3 End If If Len(msg) = 0 Then msg = "(None)" MsgBox msg, , "Selected Options"
This sample code is in the checklist.vbs file.
Download, Usage
The files mentioned in this article are contained in multichoice.zip. You can unzip them to any folder and use them however you like.
Please note that these files are provided without any guarantee or liability. Use at your own risk!